Pixel
Stylistic low-res pixelated filter
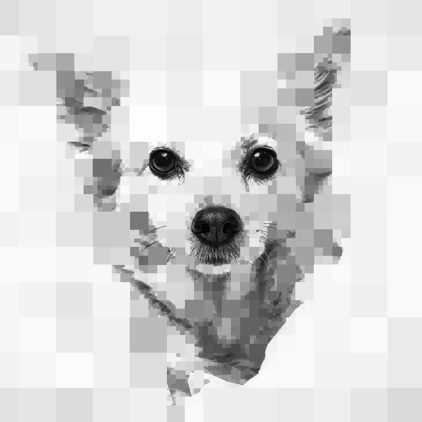
Apply filter to a photo of my dog.
#' Stylistic low-res pixelated filter
#'
#' @param img 2D matrix of intensities
#' @param t Variance threshould
#' @param klist List of voxel sizes
#' @param style Pixelation style (triangle or otherwise square)
#'
#' @return Pixelated matrix
pixel <- function(img, t=0.01, klist=c(5,10,20,40,80), style='triangle') {
mat <- img
# loop through all voxel sizes
for(k in klist) {
for(row in seq(1, nrow(mat)-k, by=k)) {
for(col in seq(1, ncol(mat)-k, by=k)) {
voxel <- mat[row:(row+k), col:(col+k)]
v <- var(as.numeric(voxel))
# if pixels within voxel have less variance than threshold, set to same intensity
if(v < t) {
set.seed(0)
if(style=='triangle') {
# set upper and lower triangles to different intensities
mat[row:(row+k), col:(col+k)][lower.tri(mat[row:(row+k), col:(col+k)])] <- sample(voxel, 1)
mat[row:(row+k), col:(col+k)][upper.tri(mat[row:(row+k), col:(col+k)])] <- sample(voxel, 1)
} else {
# set entire voxel to same intensity
mat[row:(row+k), col:(col+k)] <- sample(voxel, 1)
}
}
}
}
}
return(mat)
}
# Runner
library(jpeg)
img <- readJPEG('~/Desktop/foxxy.jpg')
# Just use red channel layer for now
mat <- img[,,1]
# Plot original image
par(mfrow=c(1,1), mar=rep(0,4))
plot.new()
lim <- par()
rasterImage(mat, lim$usr[1], lim$usr[3], lim$usr[2], lim$usr[4])
# Plot pixelated image
mat <- pixel(mat, t=0.002, style='square')
par(mfrow=c(1,1), mar=rep(0,4))
plot.new()
lim <- par()
rasterImage(mat, lim$usr[1], lim$usr[3], lim$usr[2], lim$usr[4])
Apply filter to another of my photos.
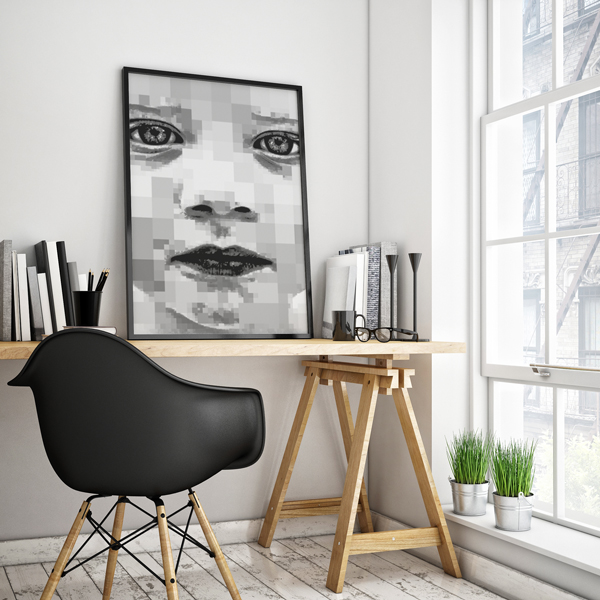